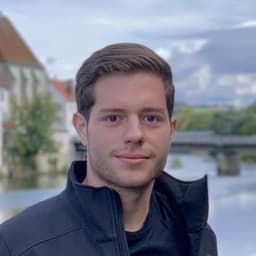
Behind the Blog: The Tech Stack that Powers My Website
I have wanted to start a blog for a while now, a place to share my thoughts and what I've learned. Even though it's a simple site for now, one thing I really want for my blog is the ability to be fully customizable. So, let's dive into how I did it:
My Blog's Tech Foundation
Since my content will primarily focus on frontend development, specifically React, I opted to use Next.js for my blog. This choice allows me to harness the power of React's Server Components while simultaneously utilizing Static Site Generation (SSG) for my blog post content. For styling, I rely on Tailwind, which enables me to customize components without writing custom CSS. Lastly, I deploy my application on Vercel.
Code Quality Matters
Before diving into writing posts or personalizing my site, I made the decision to employ some essential tools to ensure code quality. I turned to Prettier for code formatting and ESLint to spot any issues within my codebase, creating a simple Continuous Integration (CI) pipeline.
- To kick things off, I added some scripts to execute Prettier and ESLint:
"scripts": {"format": "prettier --write src","format:check": "prettier --check src","lint": "next lint --max-warnings 0"}
- Next, I incorporated additional scripts for type checking and combined them into one:
"scripts": {"typecheck": "tsc --noEmit","format": "prettier --write src","format:check": "prettier --check src","lint": "next lint --max-warnings 0","test": "npm run typecheck && npm run lint && npm run format:check"}
- Finally, I crafted a GitHub workflow for my CI:
name: CIon:push:branches:- main- devpull_request:branches:- main- devjobs:build:runs-on: ubuntu-lateststeps:- name: Checkout codeuses: actions/checkout@v2- name: Setup Nodeuses: actions/setup-node@v2with:node-version: 18- name: Install dependenciesrun: npm install- name: Run testsrun: npm test
This workflow ensures that there are no errors before merging changes into my repository, guaranteeing a robust codebase right from the start.
My Blog's Content Magic
For my blog posts, I employ MDX. MDX enables the use of JSX within markdown files, allowing me to leverage React components seamlessly. There are numerous libraries available for MDX, and after careful consideration, I opted for next-mdx-remote because it enables fetching MDX files that reside outside of my application.
As for the metadata of the blog posts, I utilize Frontmatter within the MDX files, which follows a YAML-like key/value pairing structure. Here's an example:
---title: "Behind the Blog: The Tech Stack that Powers My Website"isPublished: truepublishedOn: "2023-09-30"---## Blog post here.
Crafting Aesthetics
Previously, I mentioned that I use Tailwind for styling. Here are some of the benefits of using Tailwind:
-
No Custom CSS: With Tailwind, there's no need to write custom CSS for your application.
-
Highly Customizable: It offers a high degree of customization. For instance, I specified various padding values for different breakpoints in my container, as shown in the following example:
theme: {container: {padding: {DEFAULT: '3rem',sm: '4rem',lg: '6rem',xl: '8rem',},},}
-
Consistency: Tailwind promotes a consistent and standardized approach to styling, making it easier to maintain and scale your project.
-
Responsive Design: Tailwind includes responsive design utilities that allow you to create responsive layouts without having to write custom media queries.
In the end, there's no such thing as a perfect configuration, and undoubtedly, many aspects will evolve over time. However, I'm committed to keeping you in the loop with updates on how everything progresses. Building and maintaining a blog is not just about the tech stack or the code; it's a dynamic journey of growth and learning. So, stay tuned for more insights, tips, and the continued evolution of this blog. Thank you for joining me on this exciting adventure!